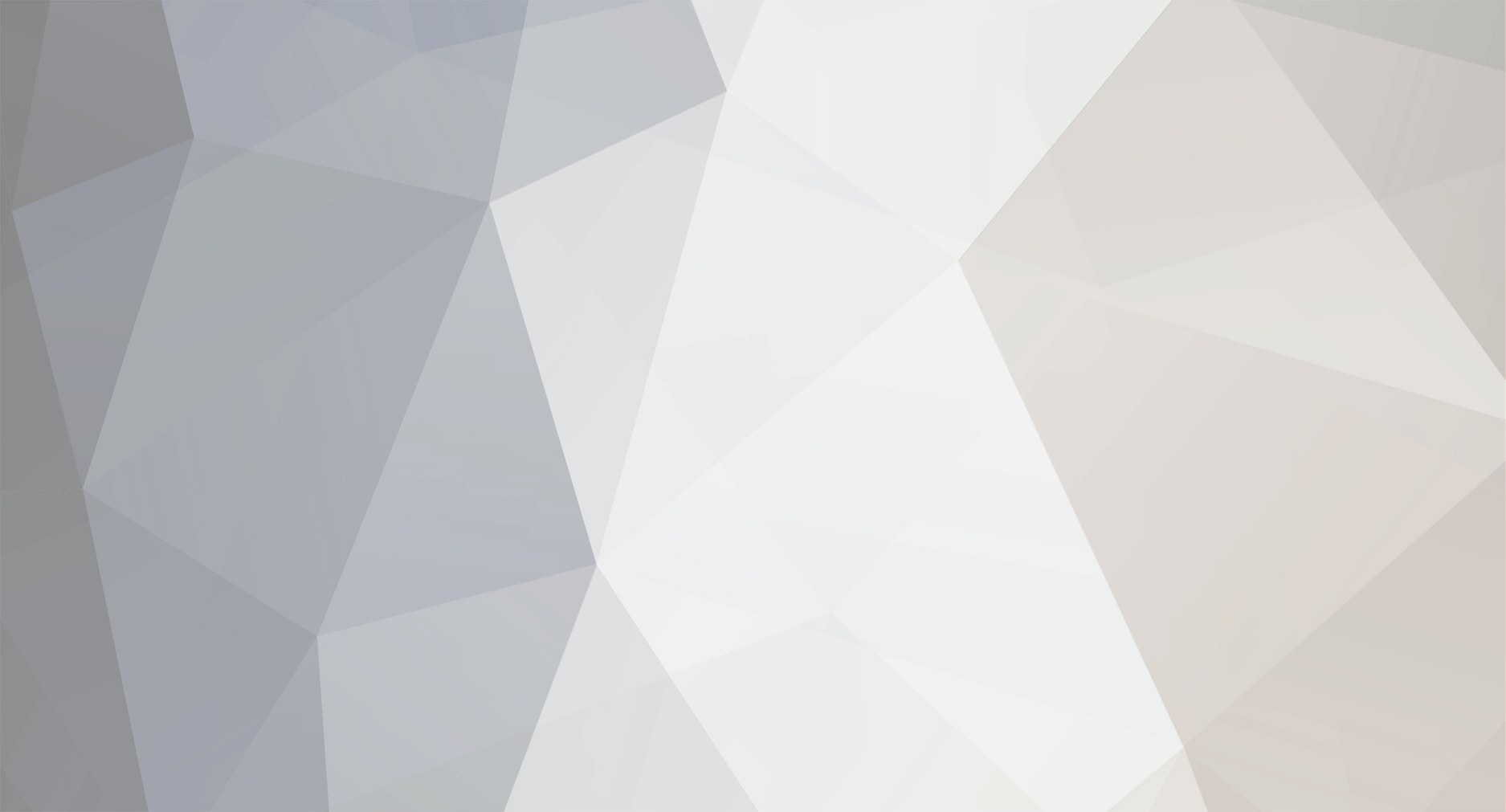
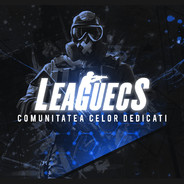
SHINEROYAL
-
Număr conținut
40 -
Înregistrat
-
Ultima Vizită
Tip conținut
Profiluri
Forumuri
Calendar
Postări postat de SHINEROYAL
-
-
Ping Faker
în Fun
- Nume plugin: Ping Faker
- Autor: MeRcyLeZZ
- Engine ( HLDS / SRCDS ) : HLDS
- Versiune: 1.4
- Informatii: Mascheaza ping-ul jucatorilor, intre 0 si 45 maxim.
- Download:
#include <amxmodx> #include <amxmisc> #include <fakemeta> new const FAKEPINGS_FILE[] = "fakepings.ini" const TASK_ARGUMENTS = 100 new cvar_enable, cvar_ping, cvar_flux, cvar_target, cvar_flags, cvar_bots, cvar_multiplier new g_enable, g_ping, g_flux, g_target, g_flags, g_bots, Float:g_multiplier, g_maxplayers new g_connected[33], g_isbot[33], g_offset[33][2], g_argping[33][3], g_hasflags[33] new g_loaded_counter, cvar_showactivity, g_pingoverride[33] = { -1, ... } new Array:g_loaded_authid, Array:g_loaded_ping public plugin_init() { register_plugin("Ping Faker", "1.4", "MeRcyLeZZ") cvar_enable = register_cvar("pingfake_enable", "1") cvar_ping = register_cvar("pingfake_ping", "45") cvar_flux = register_cvar("pingfake_flux", "5") cvar_target = register_cvar("pingfake_target", "1") cvar_flags = register_cvar("pingfake_flags", "") cvar_bots = register_cvar("pingfake_bots", "0") cvar_multiplier = register_cvar("pingfake_multiplier", "0.0") cvar_showactivity = get_cvar_pointer("amx_show_activity") g_maxplayers = get_maxplayers() register_event("HLTV", "event_round_start", "a", "1=0", "2=0") register_forward(FM_UpdateClientData, "fw_UpdateClientData") register_concmd("amx_fakeping", "cmd_fakeping", ADMIN_KICK, "<target> <ping> - Toggle fake ping override on player (-1 to disable)") g_loaded_authid = ArrayCreate(32, 1) g_loaded_ping = ArrayCreate(1, 1) load_pings_from_file() } public plugin_cfg() { // Cache CVARs after configs are loaded set_task(0.5, "event_round_start") } public event_round_start() { // Cache CVAR values g_ping = get_pcvar_num(cvar_ping) g_flux = abs(get_pcvar_num(cvar_flux)) g_target = get_pcvar_num(cvar_target) g_enable = get_pcvar_num(cvar_enable) g_bots = get_pcvar_num(cvar_bots) g_multiplier = get_pcvar_float(cvar_multiplier) // Calculate weird argument values based on target ping calculate_arguments() // Calculate them regularly if also faking ping fluctuations or if faking a multiple of the real ping remove_task(TASK_ARGUMENTS) if (g_flux || g_multiplier > 0.0) set_task(2.0, "calculate_arguments", TASK_ARGUMENTS, _, _, "b") // Cache flags new flags[6] get_pcvar_string(cvar_flags, flags, sizeof flags - 1) g_flags = read_flags(flags) // Check flags again for all players for (new id = 1; id <= g_maxplayers; id++) if (g_connected[id]) check_flags(id) } public client_authorized(id) { check_for_loaded_pings(id) check_flags(id) } public client_infochanged(id) { check_flags(id) } public client_putinserver(id) { g_connected[id] = true if (is_user_bot(id)) g_isbot[id] = true } public client_disconnect(id) { g_connected[id] = false g_isbot[id] = false g_pingoverride[id] = -1 g_hasflags[id] = 0 } public fw_UpdateClientData(id) { // Ping faking disabled? if (!g_enable) return; // Scoreboard key being pressed? if (!(pev(id, pev_button) & IN_SCORE) && !(pev(id, pev_oldbuttons) & IN_SCORE)) return; // Send fake player's pings static player, sending sending = 0 for (player = 1; player <= g_maxplayers; player++) { // Player not in game? if (!g_connected[player]) continue; // Fake latency for its target too? if (!g_target && id == player) continue; // Only do these checks if not overriding ping for player if (g_pingoverride[player] < 0) { // Is this a bot? if (g_isbot[player]) { // Bots setting disabled? if (!g_bots) continue; } else { // Bots only setting? if (g_bots == 2) continue; // Need to have specific flags? if (g_flags && !(g_hasflags[player])) continue; } } // Send message with the weird arguments switch (sending) { case 0: { // Start a new message message_begin(MSG_ONE_UNRELIABLE, SVC_PINGS, _, id) write_byte((g_offset[player][0] * 64) + (1 + 2 * (player - 1))) write_short(g_argping[player][0]) sending++ } case 1: { // Append additional data write_byte((g_offset[player][1] * 128) + (2 + 4 * (player - 1))) write_short(g_argping[player][1]) sending++ } case 2: { // Append additional data and end message write_byte((4 + 8 * (player - 1))) write_short(g_argping[player][2]) write_byte(0) message_end() sending = 0 } } } // End message if not yet sent if (sending) { write_byte(0) message_end() } } public cmd_fakeping(id, level, cid) { // Check for access flag if (!cmd_access(id, level, cid, 3)) return PLUGIN_HANDLED; // Retrieve arguments static arg[32], player, ping read_argv(1, arg, sizeof arg - 1) player = cmd_target(id, arg, CMDTARGET_ALLOW_SELF) read_argv(2, arg, sizeof arg - 1) ping = str_to_num(arg) // Invalid target if (!player) return PLUGIN_HANDLED; // Update ping overrides for player g_pingoverride[player] = min(ping, 4095) calculate_arguments() // Get player's name for displaying/logging activity static name1[32], name2[32] get_user_name(id, name1, sizeof name1 - 1) get_user_name(player, name2, sizeof name2 - 1) // Negative value means disable fakeping if (ping < 0) { // Show activity? switch (get_pcvar_num(cvar_showactivity)) { case 1: client_print(0, print_chat, "ADMIN - fake ping override disabled on %s", name2) case 2: client_print(0, print_chat, "ADMIN %s - fake ping override disabled on %s", name1, name2) } // Log activity static logdata[100], authid[32], ip[16] get_user_authid(id, authid, sizeof authid - 1) get_user_ip(id, ip, sizeof ip - 1, 1) formatex(logdata, sizeof logdata - 1, "ADMIN %s <%s><%s> - fake ping override disabled on %s", name1, authid, ip, name2) log_amx(logdata) } else { // Show activity? switch (get_pcvar_num(cvar_showactivity)) { case 1: client_print(0, print_chat, "ADMIN - fake ping override of %d enabled on %s", ping, name2) case 2: client_print(0, print_chat, "ADMIN %s - fake ping override of %d enabled on %s", name1, ping, name2) } // Log activity static logdata[100], authid[32], ip[16] get_user_authid(id, authid, sizeof authid - 1) get_user_ip(id, ip, sizeof ip - 1, 1) formatex(logdata, sizeof logdata - 1, "ADMIN %s <%s><%s> - fake ping override of %d enabled on %s", name1, authid, ip, ping, name2) log_amx(logdata) } return PLUGIN_HANDLED; } public calculate_arguments() { static player, ping, loss for (player = 1; player <= g_maxplayers; player++) { // Calculate target ping (clamp if out of bounds) if (g_pingoverride[player] < 0) { if (g_multiplier > 0.0) { get_user_ping(player, ping, loss) ping = clamp(floatround(ping * g_multiplier), 0, 4095) } else ping = clamp(g_ping + random_num(-g_flux, g_flux), 0, 4095) } else ping = g_pingoverride[player] // First argument's ping for (g_offset[player][0] = 0; g_offset[player][0] < 4; g_offset[player][0]++) { if ((ping - g_offset[player][0]) % 4 == 0) { g_argping[player][0] = (ping - g_offset[player][0]) / 4 break; } } // Second argument's ping for (g_offset[player][1] = 0; g_offset[player][1] < 2; g_offset[player][1]++) { if ((ping - g_offset[player][1]) % 2 == 0) { g_argping[player][1] = (ping - g_offset[player][1]) / 2 break; } } // Third argument's ping g_argping[player][2] = ping } } load_pings_from_file() { // Build file path new path[64] get_configsdir(path, sizeof path - 1) format(path, sizeof path - 1, "%s/%s", path, FAKEPINGS_FILE) // File not present, skip loading if (!file_exists(path)) return; // Open file for reading new linedata[40], authid[32], ping[8], file = fopen(path, "rt") while (file && !feof(file)) { // Read one line at a time fgets(file, linedata, sizeof linedata - 1) // Replace newlines with a null character to prevent headaches replace(linedata, sizeof linedata - 1, "^n", "") // Blank line or comment if (!linedata[0] || linedata[0] == ';') continue; // Get authid and ping strbreak(linedata, authid, sizeof authid - 1, ping, sizeof ping -1) remove_quotes(ping) // Store data into global arrays ArrayPushString(g_loaded_authid, authid) ArrayPushCell(g_loaded_ping, clamp(str_to_num(ping), 0, 4095)) // Increase loaded data counter g_loaded_counter++ } if (file) fclose(file) } check_for_loaded_pings(id) { // Nothing to check for if (g_loaded_counter <= 0) return; // Get steamid and ip static authid[32], ip[16], i, buffer[32] get_user_authid(id, authid, sizeof authid - 1) get_user_ip(id, ip, sizeof ip - 1, 1) for (i = 0; i < g_loaded_counter; i++) { // Retrieve authid ArrayGetString(g_loaded_authid, i, buffer, sizeof buffer - 1) // Compare it with this player's steamid and ip if (equali(buffer, authid) || equal(buffer, ip)) { // We've got a match! g_pingoverride[id] = ArrayGetCell(g_loaded_ping, i) calculate_arguments() break; } } } check_flags(id) { g_hasflags[id] = get_user_flags(id) & g_flags }
Download direct: CLICK
- Instalare: Adaugati pingfaker_shine in plugins, in config/plugins.ini adaugati pingfaker_shine.amxx
-
1
-
-
- Nume plugin: Auto reloadadmins
- Autor: shine
- Engine ( HLDS / SRCDS ) : HLDS
- Versiune: 1.0
- Informatii: auto reloadadmins setat odata la 120 secunde
- Download:
/* Plugin generated by AMXX-Studio */ #include <amxmodx> #define PLUGIN "reloadadmins" #define VERSION "1.0" #define AUTHOR "shine" public plugin_init() { register_plugin(PLUGIN, VERSION, AUTHOR) set_task(120.0,"reload",_,_,_,"b",_) } public reload( ) { server_cmd( "amx_reloadadmins" ) } /* AMXX-Studio Notes - DO NOT MODIFY BELOW HERE *{\ rtf1\ ansi\ ansicpg1252\ deff0\ deflang1033{\ fonttbl{\ f0\ fnil Tahoma;}}n\ viewkind4\ uc1\ pard\ f0\ fs16 n\ par } */
- Instalare: compilati pluginul pe amxmodx sau cum si unde vreti, adaugati linia in plugins.ini si gata.
PS: Puteti modifica secundele dupa bunul plac, set_task(120.0,"reload",_,_,_,"b",_)
-
- Nume plugin: Double jump
- Autor: Shineroyal
- Engine ( HLDS / SRCDS ) :
- Versiune: 1.0
- Informatii: Double jump setat pe flag "T" (puteti schimba dupa bunul plac)
- Download:
#include <amxmodx> #include <amxmisc> #include <engine> #define VIP_FLAG ADMIN_LEVEL_H new jumpnum[33] = 0 new bool:dojump[33] = false new cvar_vip_jumps public plugin_init() { register_plugin("MultiJump", "1.0", "shineroyal") cvar_vip_jumps = register_cvar("vip_max_jumps", "1") } public client_PreThink(id) { if(!is_user_alive(id)) { return PLUGIN_HANDLED; } new nbut = get_user_button(id) new obut = get_user_oldbutton(id) if((nbut & IN_JUMP) && !(get_entity_flags(id) & FL_ONGROUND) && !(obut & IN_JUMP)) { if(get_user_flags(id) & VIP_FLAG && get_user_team(id) == 2) { if(jumpnum[id] < get_pcvar_num(cvar_vip_jumps)) { dojump[id] = true jumpnum[id]++ return PLUGIN_CONTINUE; } } } if((nbut & IN_JUMP) && (get_entity_flags(id) & FL_ONGROUND)) { jumpnum[id] = 0 return PLUGIN_CONTINUE; } return PLUGIN_CONTINUE; } public client_PostThink(id) { if(!is_user_alive(id)) { return PLUGIN_CONTINUE; } if(get_user_flags(id) & VIP_FLAG && get_user_team(id) == 2) { if(dojump[id] == true) { new Float:velocity[3] entity_get_vector(id,EV_VEC_velocity, velocity) velocity[2] = random_float(265.0,285.0) entity_set_vector(id,EV_VEC_velocity, velocity) dojump[id] = false return PLUGIN_CONTINUE; } } return PLUGIN_CONTINUE; }
- Instalare: Compilati pluginul si adaugati.
ps: Am postat acest plugin impreuna cu bullet dmg (CLICK) mai ales pentru ownerii care doresc sa ofere putine beneficii,fara adaugarea vip-ului.
-
Dap, ai perfecta dreptate.
Merci frumos
-
- Nume plugin: RainySnowy
- Autor: - Sursa alliedmods
- Engine ( HLDS / SRCDS ) : -
- Versiune: 2.0
- Informatii: Activeaza ploaie/zapada pe server + posibilitatea random.
- Download:
#include <amxmodx> /* Choose One */ //#include <engine> #include <fakemeta> #include "ojos.inc" #define MAX_LIGHT_POINTS 3 new weather_ent new Float:g_strikedelay new g_lightpoints[MAX_LIGHT_POINTS] new g_fxbeam; new g_soundstate[33] new g_maxplayers; new g_stormintensity; public plugin_precache() { register_plugin("RainySnowy", "2.0y", "OneEyed & teame06"); register_cvar("rainysnowy", "2.0y", FCVAR_SERVER); register_cvar("weather_type", "1"); register_cvar("weather_storm", "50"); g_maxplayers = get_maxplayers(); new type = get_cvar_num("weather_type"); if(type == 3) type = random_num(0,2); switch(type) { case 1: { g_fxbeam = precache_model("sprites/laserbeam.spr"); precache_model("models/chick.mdl"); precache_sound("ambience/rain.wav"); precache_sound("ambience/thunder_clap.wav"); weather_ent = CREATE_ENTITY("env_rain") THINK("env_rain","WeatherSystem") NEXTTHINK(weather_ent,1.0) } case 2: { weather_ent = CREATE_ENTITY("env_snow"); } } } public client_putinserver(id) client_cmd(id,"cl_weather 1"); //This is only for rain. public WeatherSystem(entid) { if(entid == weather_ent) { //Is weather_storm activated? ( 0 = OFF ) -- ( 1-100 = INTENSITY ) g_stormintensity = get_cvar_num("weather_storm"); //Do our soundstate and picks random player. new victim = GetSomeoneUnworthy(); if(g_stormintensity) { //Is the delay up? if(g_strikedelay < get_gametime()) { //We got player to create lightning from? if(victim) { //Do our Lightning Technique. CreateLightningPoints(victim); } } } NEXTTHINK(weather_ent,2.0) } return PLUGIN_CONTINUE } GetSomeoneUnworthy() { new cnt, id, total[33]; for(id=1;id<g_maxplayers;id++) if(is_user_alive(id)) if(is_user_outside(id)) { total[cnt++] = id; if(!g_soundstate[id]) { g_soundstate[id] = 1; client_cmd(id, "speak ambience/rain.wav"); } } else if(g_soundstate[id]) { g_soundstate[id] = 0; client_cmd(id, "speak NULL") } if(cnt) return total[random_num(0, (cnt-1))]; return 0; } CreateLightningPoints(victim) { if(IS_VALID_ENT(g_lightpoints[0])) return 0; new ent, x, Float:tVel[3]; new Float:vOrig[3]; new Float:mins[3] = { -1.0, -1.0, -1.0 }; new Float:maxs[3] = { 1.0, 1.0, 1.0 }; new Float:dist = is_user_outside(victim)-5; //Get distance to set ents at. GET_ORIGIN(victim,vOrig) if(dist > 700.0) { //cap distance. dist = 700.0; } vOrig[2] += dist; //Create lightning bolts by spreading X entities randomly with velocity for(x=0;x<MAX_LIGHT_POINTS;x++) { ent = CREATE_ENTITY("env_sprite") SET_INT(ent,movetype,MOVETYPE_FLY) SET_INT(ent,solid,SOLID_TRIGGER) SET_FLOAT(ent,renderamt,0.0) SET_INT(ent,rendermode,kRenderTransAlpha) SET_MODEL(ent,"models/chick.mdl") SET_VECTOR(ent,mins,mins) SET_VECTOR(ent,maxs,maxs) tVel[0] = random_float(-500.0,500.0); tVel[1] = random_float(-500.0,500.0); tVel[2] = random_float((dist<=700.0?0.0:-100.0),(dist<=700.0?0.0:50.0)); SET_VECTOR(ent,origin,vOrig) SET_VECTOR(ent,velocity,tVel) g_lightpoints[x] = ent; } emit_sound(ent, CHAN_STREAM, "ambience/thunder_clap.wav", 1.0, ATTN_NORM, 0, PITCH_NORM) set_task(random_float(0.6,2.0),"Lightning",victim); return 1; } // Creating a beam at each entity consecutively. // Player has 1 in 1000 chance of getting struck ! public Lightning(victim) { new x, a, b, rand; new endpoint = MAX_LIGHT_POINTS-1; while(x < endpoint) { a = g_lightpoints[x]; b = g_lightpoints[x+1]; x++ if(x == endpoint) { rand = random_num(1,1000); //One unlucky son of a bish. if(rand == 1) { b = victim; FAKE_DAMAGE(victim,"Lightning",100.0,1); } } CreateBeam(a,b); } for(x=0;x<MAX_LIGHT_POINTS;x++) if(IS_VALID_ENT(g_lightpoints[x])) REMOVE_ENTITY(g_lightpoints[x]) //Set up next lightning. if(g_stormintensity > 100) { set_cvar_num("weather_storm", 100); g_stormintensity = 100; } new Float:mins = 50.0-float(g_stormintensity/2); new Float:maxs = 50.0-float(g_stormintensity/3); g_strikedelay = get_gametime() + random_float(mins, maxs); } //return distance above us to sky Float:is_user_outside(id) { new Float:origin[3], Float:dist; GET_ORIGIN(id, origin) dist = origin[2]; while (POINTCONTENTS(origin) == -1) origin[2] += 5.0; if (POINTCONTENTS(origin) == -6) return (origin[2]-dist); return 0.0; } CreateBeam(entA, entB) { message_begin( MSG_BROADCAST, SVC_TEMPENTITY ); write_byte( 8 ); write_short( entA ); write_short( entB ); write_short( g_fxbeam ); write_byte(0); //start frame write_byte(10); //framerate write_byte(5); //life write_byte(8); //width write_byte(100); //noise write_byte(255); //red write_byte(255); //green write_byte(255); //blue write_byte(255); //brightness write_byte(10); //scroll speed message_end(); }
- Instalare: Compilati sursa,numiti plugin-ul si adaugati linia in plugins.ini dupa ce faceti upload in plugins. Adaugati ojos.inc scripting/include/ . Pentru a descarca ojos.inc CLICK
Cvars:
- weather_type < 0 | 1 | 2 | 3 > - ( 0 = OFF | 1 = RAIN | 2 = SNOW | 3 = RANDOM ) - weather_storm < 0 - 100 > - ( 0 = OFF | 1-100 = INTENSITY ) - Only works when its raining.
-
- Nume plugin: bullet damage
- Autor: -
- Engine ( HLDS / SRCDS ) : hlds
- Versiune: 1.0
- Informatii: Puteti seta flag-ul dupa bunul plac. Default este pe flag "t"
- Download:
#include <amxmodx> new g_HudSyncObj; public plugin_init() register_event("Damage", "Damage", "b", "2!0", "3=0", "4!0"); public plugin_cfg() g_HudSyncObj = CreateHudSyncObj(); public Damage(Cl) { static Attacker; Attacker = get_user_attacker(Cl); if (get_user_flags(Attacker) & ADMIN_LEVEL_H) { set_hudmessage(0, 100, 200, -1.0, 0.55, 2, 0.1, 4.0, 0.02, 0.02, 7); ShowSyncHudMsg(Attacker, g_HudSyncObj, "%d^n", read_data(2)); } }
- Instalare: Compilati sursa si numiti plugin'ul dupa bunul plac.
-
Nume plugin: Evidenta Log
- Autor: Anakin
- Engine ( HLDS / SRCDS ) : HLDS
- Versiune: 1.0
- Informatii: Acest plugin creaza un document log.txt in addons / amxmodx / configs unde vor aparea IP/SteamID playerilor conectati pe server.
- Download:
#include <amxmodx> #include <amxmisc> #define PLUGIN "Log IP" #define VERSION "1.0" #define AUTHOR "Anakin" new toggle; public plugin_init() { register_plugin(PLUGIN, VERSION, AUTHOR); toggle = register_cvar("ip_log","1"); } public client_connect(id) { if(get_pcvar_num(toggle) != 1) return; IP_Log(id); } IP_Log(id) { new szFile[128]; get_configsdir(szFile,127); formatex(szFile,127,"%s/ip_log.txt",szFile); if(!file_exists(szFile)) { write_file(szFile,"Ips Connected",-1); write_file(szFile," ",-1) } new name[32],ip[32],sztime[54],szLog[256], szAuthID[32]; get_user_name(id,name,31); get_user_ip(id,ip,31); get_user_authid(id, szAuthID, charsmax(szAuthID)); get_time("%m.%d.%Y -- %H:%M:%S",sztime,53); formatex(szLog,255,"Player: %s IP: %s SteamID: %s Date: %s",name,ip, szAuthID, sztime); write_file(szFile,szLog,-1); }
- Instalare: Compilati sursa si numiti plugin'ul dupa bunul plac.
[Plugin] VIP Clasic 1.7 - NEW VERSION
în Fun
Postat
Este foarte simplu de adeugat, intra pe canalul de suport tehnic ts3 in cel mai rau caz... totusi repet.. este simplu